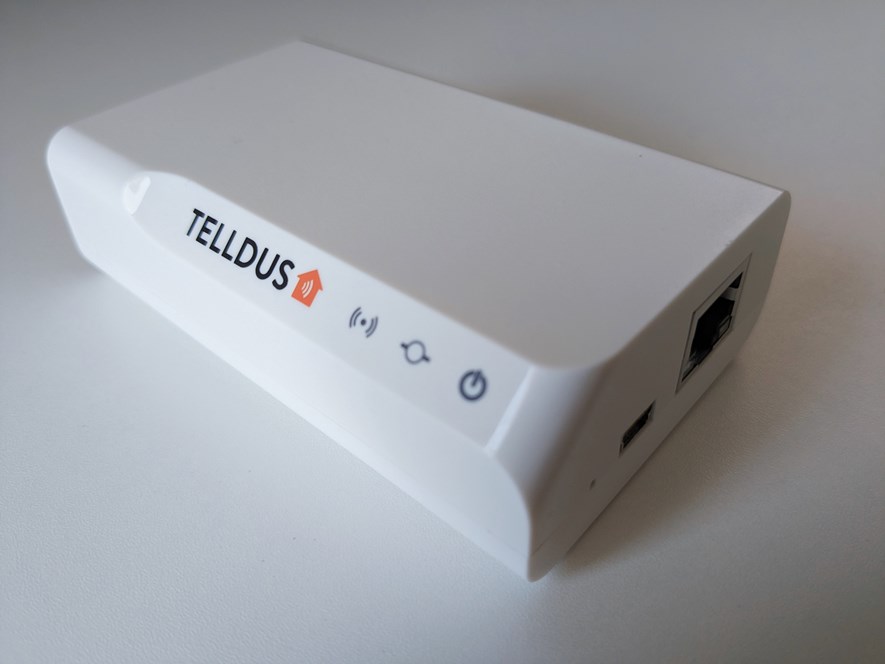
Telldus Tellstick: Simple Scheduler with Lua
Because scheduling is...fun
Home automation is nothing I personally go overboard with, but sometimes it's convenient to have lights turn on and off by a schedule. Several years ago, I bought a Telldus Tellstick ZNet Lite V2 for this exact purpose. While the device could do more, I have simply used it to control smart plugs that turn on or off depending on the current time as well as the times for sunrise and sunset. However, I recently discovered that scheduling has been remotely disabled by the manufacturer. While you used to be able to use an app to set up a schedule, the app now tells you that using a schedule requires a premium subscription. Turning the lights on or off manually using the app is still possible but since I purchased the device to use its scheduler, this change pretty much defeats the purpose of owning the device in the first place. Luckily, the Tellstick does offer the possibility to run custom Lua scripts, which you can write and upload yourself. Let's write us a scheduler!
What we want
We simply want a script where we can set up a predefined list of times, such as 06:00, 10:00, etc., and a property for each entry to tell the device if it should be on or off at that point. So a possible solution would be to check where the current time fits into the list of times, then turn the device on or off if needed, and then sleep for a minute before performing the check again. This would then loop indefinitely.
Alternatively, the script could determine the time until next change, and sleep until then, wake up, switch on/off the device, calculate time until next change, sleep until then, etc. But I will leave that solution as an excercise to the reader.
Lua scripting
Scripts in the Lua programming language can be uploaded to the device to be run inside a sandbox. Neat idea, but official Lua documentation from Telldus is a bit hard to find. In our case however we don't need to do much, just write a simple scheduler, so the examples provided by Telldus will serve us well enough.
Aside: There is some old documentation available at Read the Docs but strangely, if you select the latest version, it's simply empty. It seems the section was removed in this Git commit and the Python plugin documentation extended. Possíbly the manufacturer would prefer we use Python instead of Lua? But I digress...
After looking at the examples, I found the basic "Telldus specific" things we need in order to cobble together want we want. Here are some useful statements to demonstrate:
local deviceManager = require "telldus.DeviceManager" -- Import the DeviceManager module
local device = deviceManager:findByName("myDeviceName") -- Get the device
device:command("turnon", nil, "myLuaScript") -- Turn on the device
sleep(60*1000) -- Sleep for a minute
device:command("turnoff", nil, "myLuaScript") -- Turn off the device
So we can now use the commands above and add our scheduler logic using Lua. Of course, some basic Lua knowledge is helpful, but as it turns out, not required - Lua is a rather small language and relatively easy to pick up. DuckDuckGo is your friend!
Download and make it yours
To create the script, you can use this as a starting point: time-schedule.lua. Download the file and open it in a text editor. At the top of the file, you will find a few things to adapt first:
1. Enter the name of the name of the device you want to turn on and off. If you have several devices to control with the same schedule, you can set up a group (only available in the Telldus Live web interface, not the app) and enter the group name below.
local deviceName = "EnterDeviceNameHere"
2. Define time intervals. Each entry has the properties
local intervals = {
{ hour=06, min=00, on=true },
{ hour=08, min=00, on=false },
{ hour=16, min=00, on=true },
{ hour=22, min=00, on=false }
}
Upload and run
After your changes, you will need to upload the file to the device - by means of copy and paste.
In order to access the management page of the device, you will need its local IP on the network. The easiest way is probably to use the Telldus app. In the app, open the menu and select the Tellstick. The local IP should show up next to the device. If you don't have the app, you can probably figure out the IP by logging on to your network router and look for connected devices.
Once you have the IP (which might look something like this:
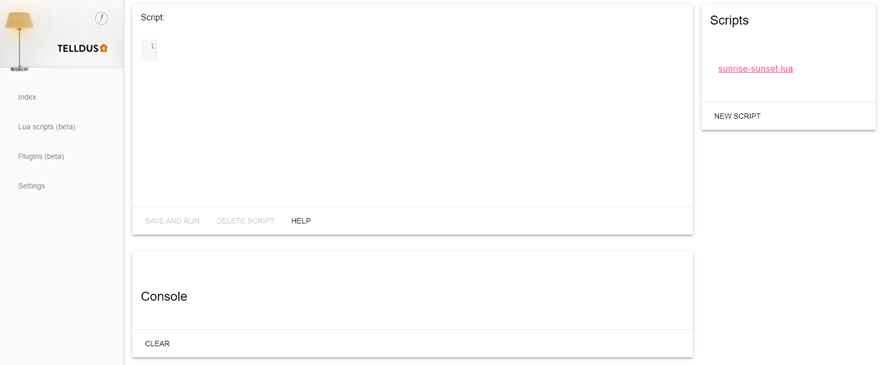
On the management page, open "Lua scripts", click "New script". Type in a name for the script, paste the script you created previously, and hit "Save and run".
The script now starts and will always be running from this point. It will also be started automatically if the device was turned off and is turned on again.
Make it dynamic
If you need a script that uses different times depending on sunset and sunrise, you can try this: sunrise-sunset.lua.
Note that the times in the file are hard coded for a specific location, so you may need to copy-paste-adapt the times for your own location.
Happy coding!
0 Comments
Subscribe to new comments by RSS